最近在刷树相关的LeetCode题目,想在本地调试的话比较麻烦,因此写了同LeetCode里基于数组转树结构的WebStorm代码模版,具体思路就是创建一个树结点队列,数组也当做队列来用,首先把head头节点创建出并加入到树结点队列中,然后需要判断当前构建树的数组是否还有值,存在的话取队列中的第一个结点,当作父节点,接下来取数组中的第一个元素,当前的数组中取出的元素不能为null空,新建结点;否则不新建结点,最后当array数组为空的时候返回head。
如何创建WebStorm代码模版,请见我的另一篇文章使用头插法尾插法合并两个顺序链表
使用本文方法对数组[1, 2, 3, null, 4, 5, 6, 7],转为二叉树结构后,如下图所示:
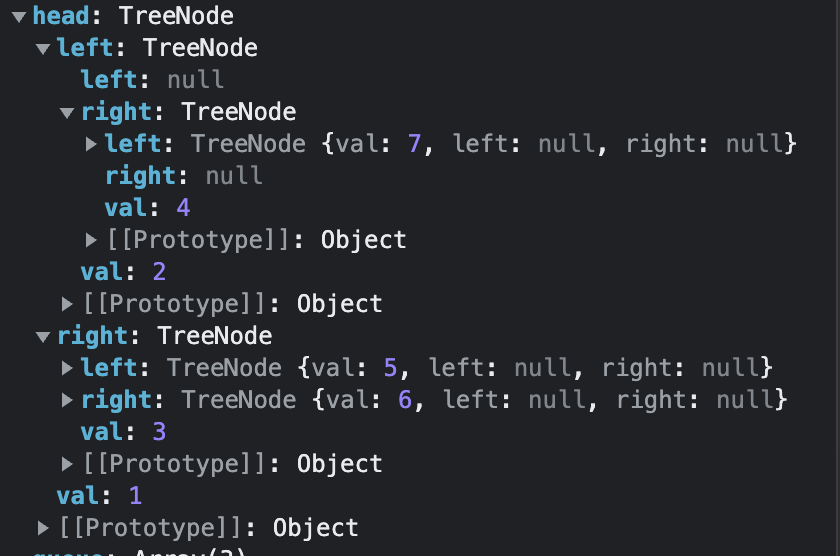
数组转二叉树的代码如下,也相当于是给你一个数组,如何转为二叉树的算法题解。
function TreeNode(val, left, right) { this.val = (val === undefined ? 0 : val) this.left = (left === undefined ? null : left) this.right = (right === undefined ? null : right) }
const getTreeFromArray = (array) => { if (!array || !array.length) { return null; }
const queue = []; const head = new TreeNode(array.shift()); queue.push(head);
while (array.length) { const parent = queue.shift(); let curVal = array.shift() if (typeof curVal === 'number') { const node = new TreeNode(curVal); parent.left = node; queue.push(node); } curVal = array.shift() if (typeof curVal === 'number') { const node = new TreeNode(curVal); parent.right = node; queue.push(node); } } return head; }
getTreeFromArray([1, 2, 3, null, 4, 5, 6, 7])
|